In Java, a constructor is a special method within a class that is responsible for initializing the objects of that class. When you create an instance of a class using the new keyword, a constructor is automatically called to set up the initial state of the object.
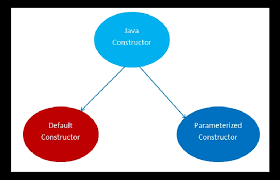
Constructors have the following characteristics:
- Name: The constructor’s name is always the same as the class name. It does not have a return type, not even void.
- Initialization: The primary purpose of a constructor is to initialize the object’s state, typically by assigning initial values to the object’s instance variables.
- Access modifier: Constructors can have access modifiers such as public, protected, private, or package-private (no explicit modifier). The access modifier determines from where the constructor can be accessed.
- Overloading: Like other methods in Java, constructors can be overloaded. This means you can have multiple constructors in the same class, each with a different set of parameters. Overloading allows you to create objects with different initial states.
Here’s an example of a simple class with a constructor:
public class MyClass {
private int value;
// Default constructor with no parameters
public MyClass() {
value = 0; // Default value
}
// Constructor with a parameter
public MyClass(int initialValue) {
value = initialValue;
}
// Other methods and members can follow...
}
In this example, we have two constructors. The first one is a default constructor with no parameters, which sets the value variable to 0 when an object is created without providing an initial value explicitly. The second constructor takes an int parameter and assigns it to the value variable.
You can create objects using these constructors as follows:
MyClass obj1 = new MyClass(); // Uses the default constructor, value = 0
MyClass obj2 = new MyClass(42); // Uses the parameterized constructor, value = 42
Remember that if you don’t explicitly define any constructor in your class, Java provides a default constructor with no parameters, which initializes instance variables to their default values (0, null, or false, depending on the data type). However, once you define any constructor in the class, the default constructor won’t be automatically provided, so you’ll have to explicitly define it if needed.